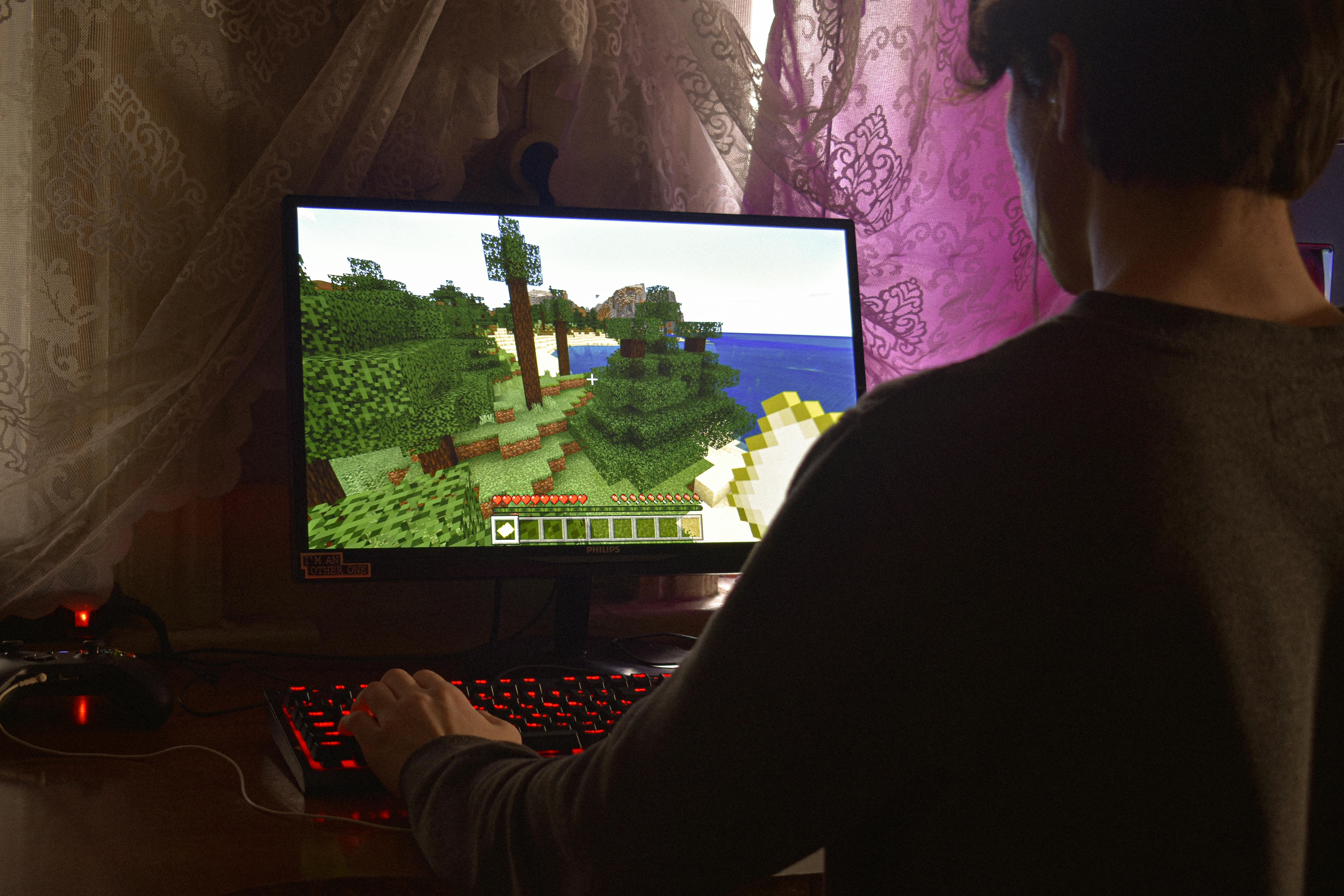
本文探讨了如何使用 Amazon Q Developer 根据结构化的提示词,直接生成一个贪吃蛇游戏原型,并剖析了其背后人工智能的思考和迭代完善过程,展示了人工智能能快速进行游戏原型创作的巨大潜力。
原文出处来自作者于 2024 年 9 月在 community.aws 发表的技术文章:
“From Concept to Playable in Seconds: Creating the Greedy Snake Game with Amazon Q Developer”
概述
在我之前的“Amazon Bedrock 实践:零基础创建贪吃蛇游戏”的文章中,我展示了如何使用 Amazon Bedrock 和结构化的文本提示词,直接生成贪吃蛇游戏原型的完整过程。文章发表后,许多开发人员反馈说深受这篇文章的启发,并好奇地询问 Amazon Q Developer 是否也能够类似这样来直接生成贪吃蛇游戏原型。这就是我将在本文中具体和各位开发者探讨的内容。
贪吃蛇是一款风靡了几代人的老式游戏。游戏目标很简单:
通过控制一条不断增长的小蛇,引导它吃下食物,同时避免撞到游戏界面的边界或蛇身。小蛇吃下越多食物,身体就会越长,游戏难度也就越大,需要玩家迅速反应和具备全局策略思维。
接下来,让我们看看 Amazon Q Developer 通过结构化的自然语言提示词,来直接生成完整贪吃蛇游戏原型代码的非凡能力。
提示词
我将使用之前那篇关于 Amazon Bedrock 生成贪吃蛇原型代码的博客文章中,相同的结构化提示词,来与 Amazon Q Developer 进行交互;这样各位读者将可以观察到:Amazon Q Developer 和 Amazon Bedrock 在整个代码生成过程中的具体差异和区别。
以下是我用于生成贪吃蛇游戏原型代码的提示词:
“Write a short and high-quality python script for the following task, something a very skilled python expert would write. You are writing code for an experienced developer so only add comments for things that are non-obvious. Make sure to include any imports required.
NEVER write anything before the ```python``` block. After you are done generating the code and after the ```python``` block, check your work carefully to make sure there are no mistakes, errors, or inconsistencies.
If there are errors, list those errors in tags, then generate a new version with those errors fixed. If there are no errors, write "CHECKED: NO ERRORS" in tags.
Here is the task: write a greedy snake game.
Double check your work to ensure no errors or inconsistencies.”
如上所述,整段结构化的提示词提供了对游戏的主要功能、需使用的库、以及其他实现细节的详细要求。在提示词中提供这种程度的具体细节描述,对于获得高质量的代码输出至关重要。
开发特性 /dev
如下图所示,在 Amazon Q Developer 聊天对话框中,我在输入提示词描述之前,先输入了 "/dev"。
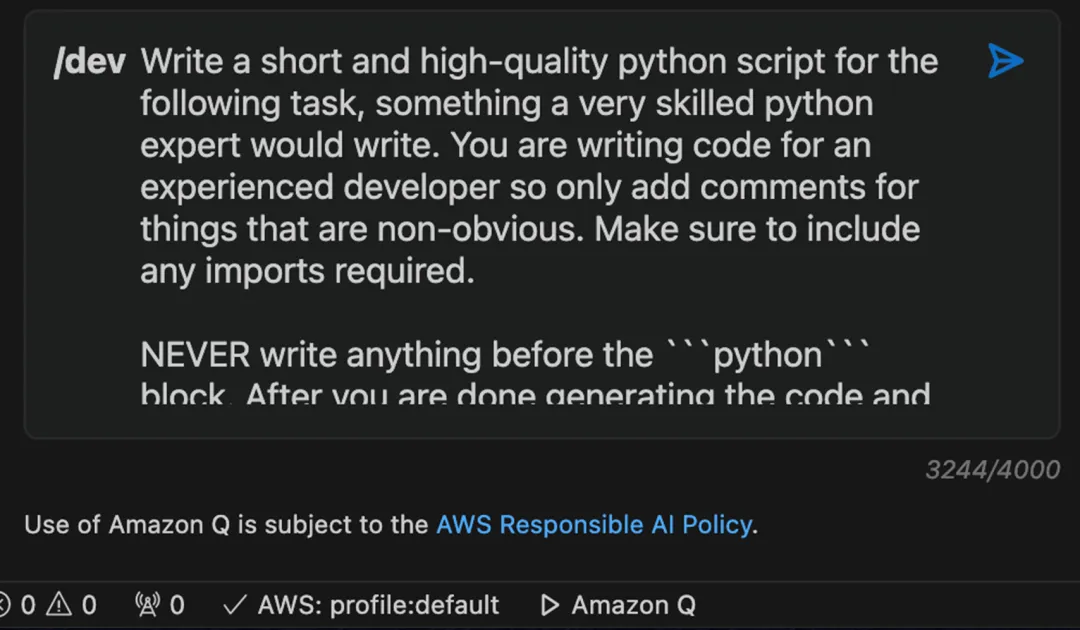
在 Amazon Q Developer 的上下文中,"/dev" 是一个特殊命令,用于调用 Amazon Q Developer Agent 进行软件开发,而不是指 Linux 系统中的目录。
您可以参考 Amazon Q Developer Agent 文档,以了解详细信息。
与 Amazon Q Developer 互动
以下截图展示了与 Amazon Q Developer 的聊天互动界面。我要求其为贪吃蛇游戏原型生成高质量的 Python 脚本,其确认了该请求,并告知我生成代码可能需要的时间(几分钟)。
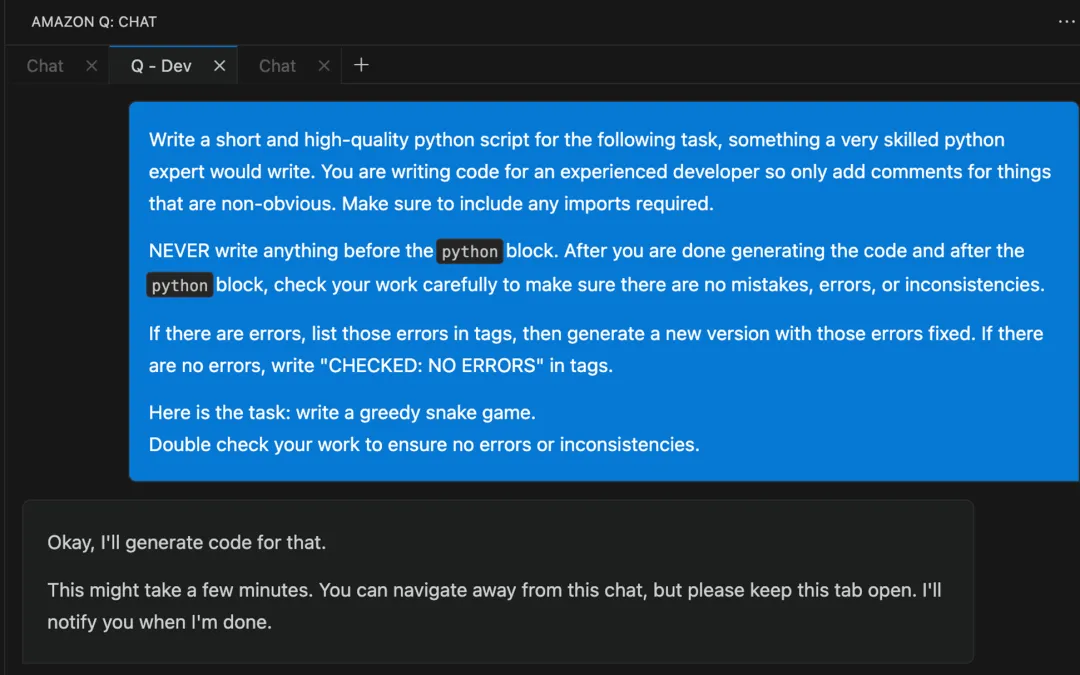
数分钟后,Amazon Q Developer 以思考过程汇总的形式作出了回应,这其实也体现了其背后大型语言模型的思考过程,如下图所示。
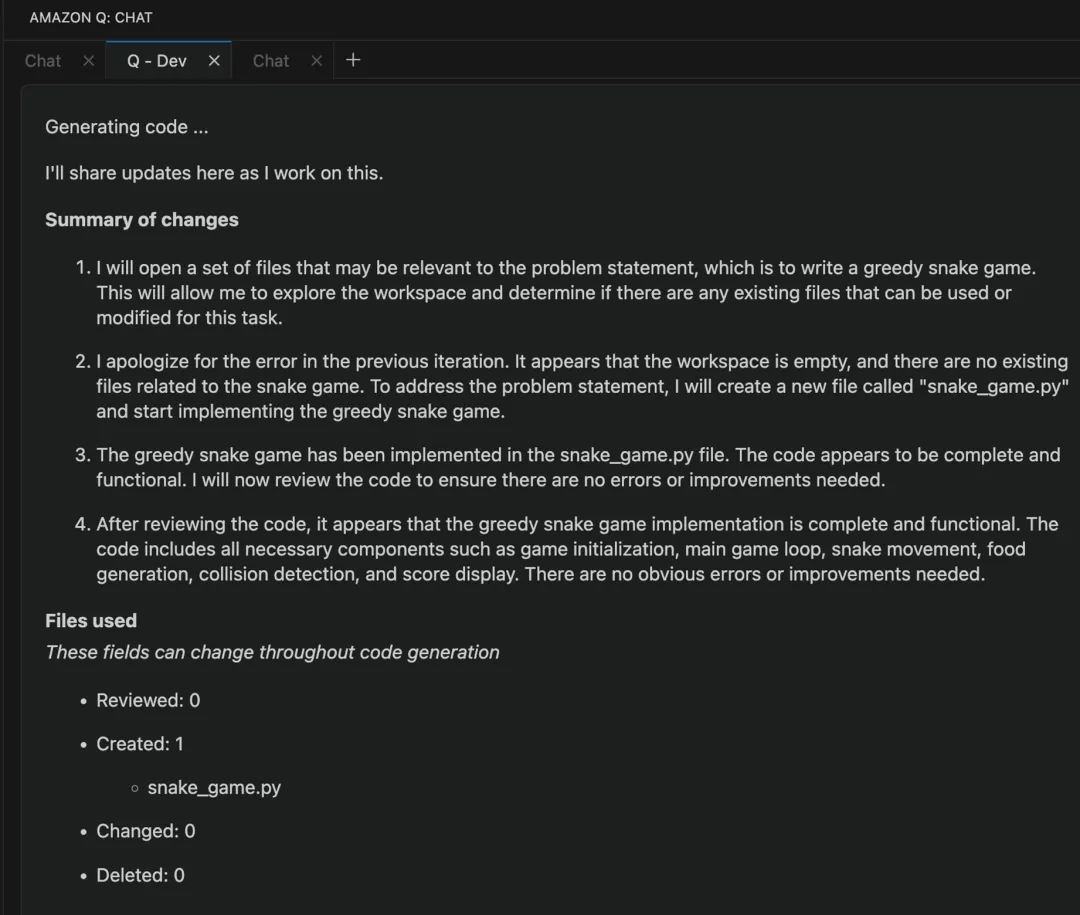
让我们来一起梳理 Amazon Q Developer 的思考过程。
根据 Amazon Q Developer 的以上汇总摘要,其创建一款贪食蛇游戏原型经历了以下步骤:
首先,它计划打开与编写贪食蛇游戏的问题陈述相关的现有本地代码,以探索工作空间并确定是否有可以重用或修改的代码。
接着,它发现因为工作区是空的,没有与贪吃蛇游戏相关的代码文件,需要自己重新编写。为了解决这个问题,它决定创建一个名为 "snake_game.py" 的 Python 新文件,并准备从头开始实现贪食蛇游戏原型代码。
贪食蛇游戏原型代码,随后在 "snake_game.py" 的 Python 文件中被它实现了,它还声明:代码已经完成并能正常运行了。
然后,它对代码进行了仔细地审阅。审阅完成后,它确认了贪食蛇游戏原型的代码已经完整实现,并已经能够正常运行了。它还特别提及:代码已经包括了所有必需的组件,例如游戏初始化、主游戏循环、蛇形移动、食物生成、碰撞检测和得分显示等等。
图中底部的 "Files used" 部分,总结了代码生成过程中执行的文件操作,供我们参考。
输出完整的贪吃蛇游戏原型代码
以下代码即由 Amazon Q Developer 完整生成的游戏原型代码。
import pygame
import random
# Initialize Pygame
pygame.init()
# Set up the game window
width, height = 640, 480
window = pygame.display.set_mode((width, height))
pygame.display.set_caption("Greedy Snake")
# Colors
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
# Snake properties
snake_block = 20
snake_speed = 15
# Initialize clock
clock = pygame.time.Clock()
# Font for score display
font = pygame.font.SysFont(None, 50)
def draw_snake(snake_list):
for block in snake_list:
pygame.draw.rect(window, GREEN, [block[0], block[1], snake_block, snake_block])
def game_loop():
game_over = False
game_close = False
# Starting position of the snake
x1 = width // 2
y1 = height // 2
# Initial movement
x1_change = 0
y1_change = 0
# Initialize snake
snake_list = []
length_of_snake = 1
# Generate first food
foodx = round(random.randrange(0, width - snake_block) / snake_block) * snake_block
foody = round(random.randrange(0, height - snake_block) / snake_block) * snake_block
while not game_over:
while game_close:
window.fill(BLACK)
message = font.render("You Lost! Press Q-Quit or C-Play Again", True, RED)
window.blit(message, [width // 6, height // 3])
pygame.display.update()
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_q:
game_over = True
game_close = False
if event.key == pygame.K_c:
game_loop()
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
x1_change = -snake_block
y1_change = 0
elif event.key == pygame.K_RIGHT:
x1_change = snake_block
y1_change = 0
elif event.key == pygame.K_UP:
y1_change = -snake_block
x1_change = 0
elif event.key == pygame.K_DOWN:
y1_change = snake_block
x1_change = 0
# Check if snake hits the boundaries
if x1 >= width or x1 < 0 or y1 >= height or y1 < 0:
game_close = True
x1 += x1_change
y1 += y1_change
window.fill(BLACK)
pygame.draw.rect(window, RED, [foodx, foody, snake_block, snake_block])
snake_head = [x1, y1]
snake_list.append(snake_head)
if len(snake_list) > length_of_snake:
del snake_list[0]
# Check if snake hits itself
for segment in snake_list[:-1]:
if segment == snake_head:
game_close = True
draw_snake(snake_list)
# Display score
score = font.render(f"Score: {length_of_snake - 1}", True, WHITE)
window.blit(score, [0, 0])
pygame.display.update()
# Check if snake eats food
if x1 == foodx and y1 == foody:
foodx = round(random.randrange(0, width - snake_block) / snake_block) * snake_block
foody = round(random.randrange(0, height - snake_block) / snake_block) * snake_block
length_of_snake += 1
clock.tick(snake_speed)
pygame.quit()
quit()
if __name__ == "__main__":
game_loop()
以下截图展示了以 Amazon Q Developer 生成的贪吃蛇游戏原型代码片段为背景,前景为该游戏代码运行时的截图:
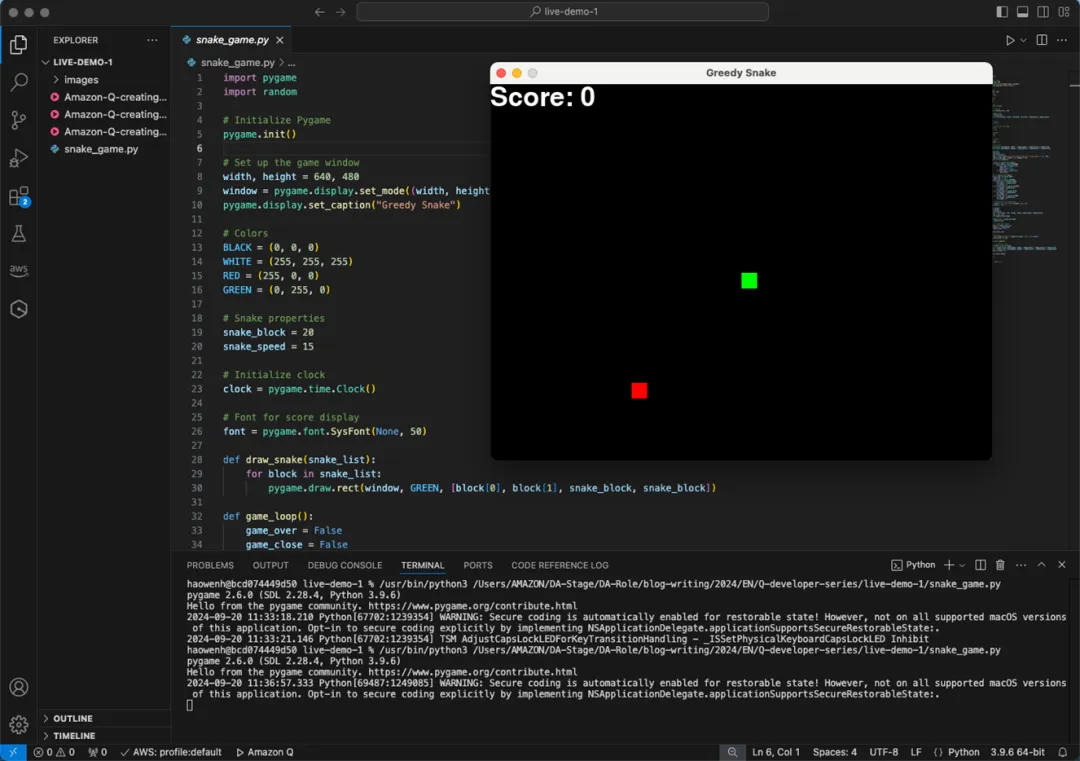
在深入了解 Amazon Q Developer 实现的游戏原型代码之前,理解 Pygame 在游戏开发中的作用是非常重要的。Pygame 是一组专门用于编写多媒体应用程序(包括游戏)的 Python 模块。它提供了丰富的功能,从处理图形、声音,到管理用户输入、游戏事件等等。
上述代码是使用 Pygame 库编写的经典贪吃蛇游戏原型的 Python 实现。
其主要功能包括:设置游戏窗口、初始化蛇和位置、生成食物、处理用户输入以控制蛇身移动、检测与边界或蛇身是否发生碰撞、更新游戏状态,以及在屏幕上渲染游戏元素等等。游戏将持续进行,直到蛇与边界或自身发生碰撞,此时玩家可以选择退出或重新启动游戏。
不过,如果你是一个游戏新手,以上 AI 生成的游戏原型代码运行后,蛇移动的速度可能会过快,从而让游戏初级玩家无法掌控。
因此,我接着给 Amazon Q Developer 又安排了一个新任务:
调整蛇奔跑的速度。
调整蛇的奔跑速度
以下是我拟提交给 Amazon Q Developer 的交互对话框,从而可以调整贪吃蛇游戏中蛇奔跑速度的英文提示词:
“The code works, but the speed of the snake is too fast. I'm just a beginner at the game, could you adjust the game's speed for me?”
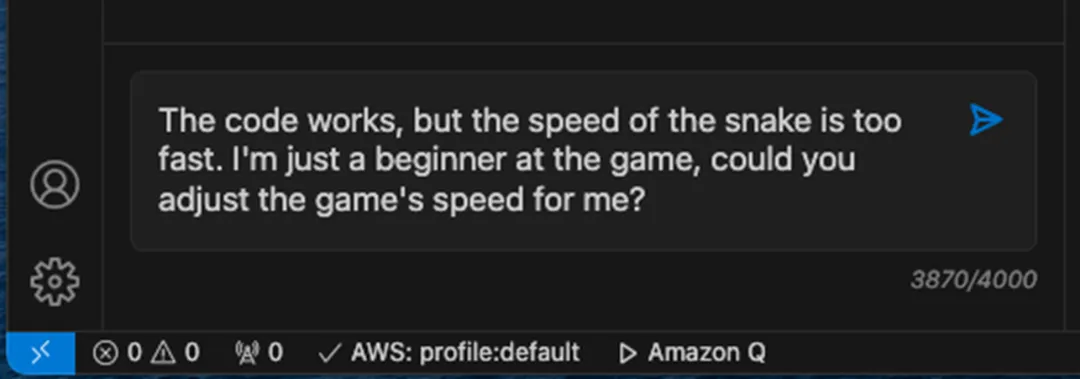
在提交提示词后,Amazon Q Developer 迅速作出回应,截图如下所示:
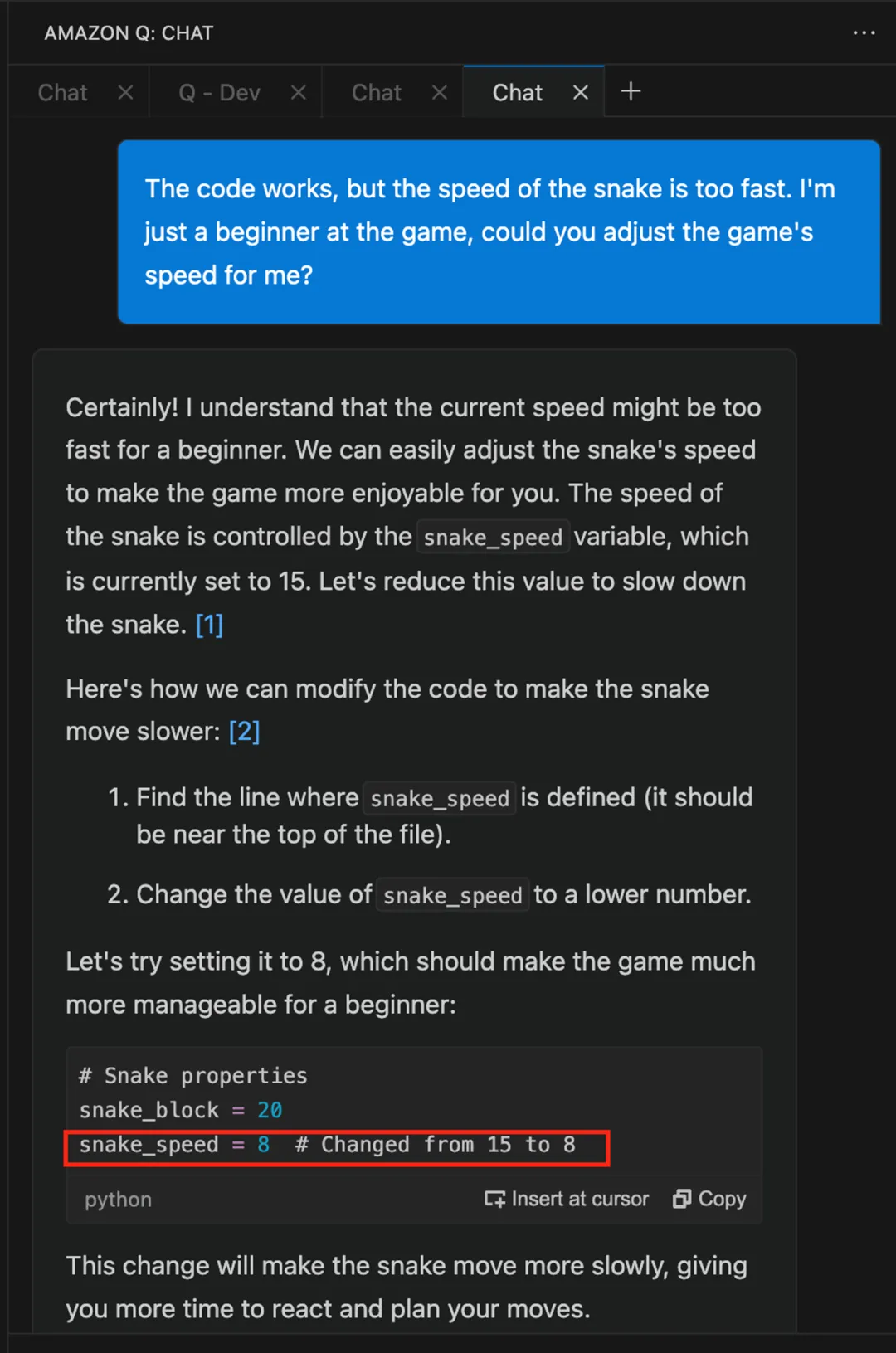
以上截图展示了一段我和 Amazon Q Developer 的聊天对话记录。
我要求对贪吃蛇游戏的代码进行调整,并表示当前的速度对于初学者来说太快了。
Amazon Q Developer 的回复,解释了如何将初始值为 15 的 snake_speed 变量修改为较低的 8。这样蛇的移动速度就会减慢,游戏对初级玩家来说会更加易于控制。底部的代码片段显示了将 snake_speed 从 15 改为 8,并添加了注释说明这一调整。
你可以按照上述建议进行操作,只需要修改其中一行代码,如下所示:
snake_speed = 8
把新修改后的代码运行起来。你会发现:蛇奔跑的速度比之前慢多了,即使是游戏初级玩家也可以自由地控制蛇的运行啦!
小结
本文展示了 Amazon Q Developer 在根据自然语言的结构化提示词,生成游戏原型代码方面的卓越潜力。
生成的代码体现了 Amazon Q Developer 以及其背后的大模型,理解复杂需求、利用流行库以及根据用户反馈进行迭代改进的巨大潜力。通过调整蛇的速度,其展现了对个性化游戏体验的适应性探索,而整个思维过程的汇总提供了其宝贵的见解,增强了人类对 AI 系统的透明度和信任度。
随着 AI 技术的不断进步,像 Amazon Q Developer 这样的 AI 工具有可能彻底改变软件开发领域,加速应用程序或游戏的原型制作和迭代周期,并极大地促进创新。虽然本文展示的贪吃蛇游戏只是一个较简单的案例,但 Amazon Q Developer 展示出来的能力,为其在更复杂的应用程序开发方向开辟了令人兴奋的无限可能。
评论