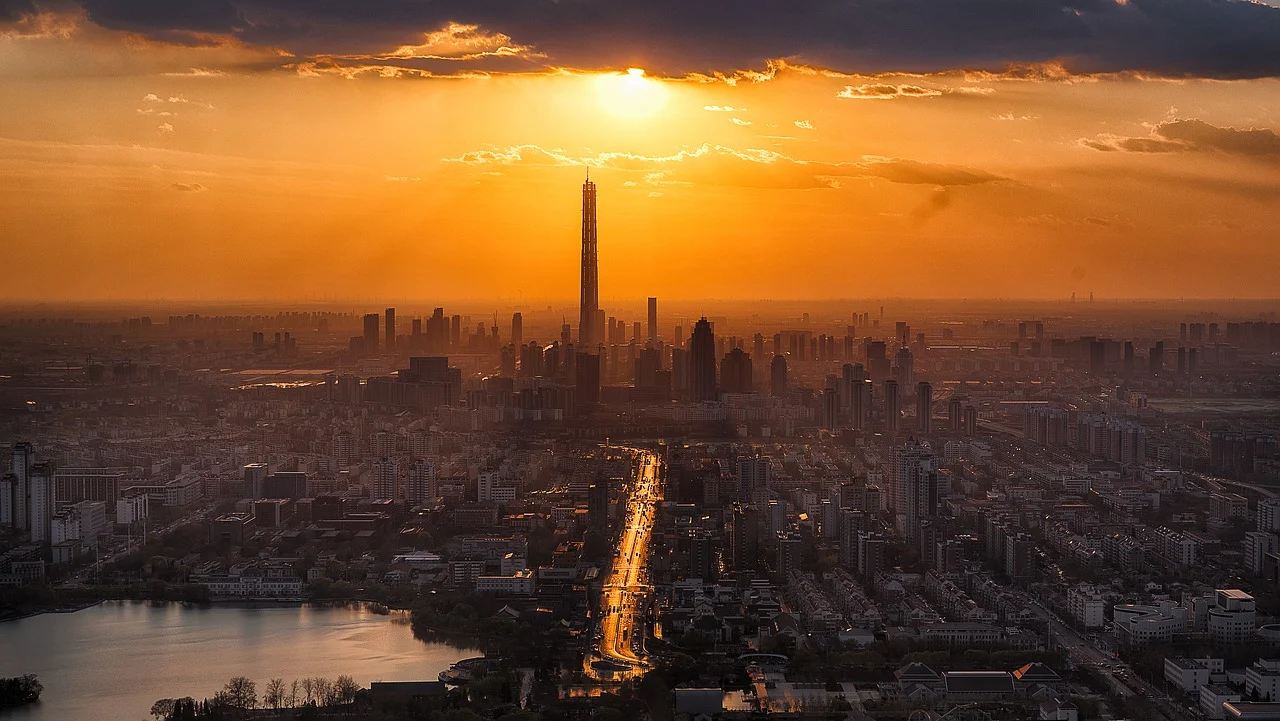
本文,我们将通过 Python 语言包,来构建一些机器学习模型。
构建机器学习模型的模板
该 Notebook 包含了用于创建主要机器学习算法所需的代码模板。在 scikit-learn 中,我们已经准备好了几个算法。只需调整参数,给它们输入数据,进行训练,生成模型,最后进行预测。
1.线性回归
对于线性回归,我们需要从 sklearn 库中导入 linear_model。我们准备好训练和测试数据,然后将预测模型实例化为一个名为线性回归 LinearRegression 算法的对象,它是 linear_model 包的一个类,从而创建预测模型。之后我们利用拟合函数对算法进行训练,并利用得分来评估模型。最后,我们将系数打印出来,用模型进行新的预测。
# Import modules
from sklearn import linear_model
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted_variable
x_test = test_dataset_precictor_variables
# Create linear regression object
linear = linear_model.LinearRegression()
# Train the model with training data and check the score
linear.fit(x_train, y_train)
linear.score(x_train, y_train)
# Collect coefficients
print('Coefficient: \n', linear.coef_)
print('Intercept: \n', linear.intercept_)
# Make predictions
predicted_values = linear.predict(x_test)
2.逻辑回归
在本例中,从线性回归到逻辑回归唯一改变的是我们要使用的算法。我们将 LinearRegression 改为 LogisticRegression。
# Import modules
from sklearn.linear_model import LogisticRegression
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted_variable
x_test = test_dataset_precictor_variables
# Create logistic regression object
model = LogisticRegression()
# Train the model with training data and checking the score
model.fit(x_train, y_train)
model.score(x_train, y_train)
# Collect coefficients
print('Coefficient: \n', model.coef_)
print('Intercept: \n', model.intercept_)
# Make predictions
predicted_vaues = model.predict(x_teste)
3.决策树
我们再次将算法更改为 DecisionTreeRegressor:
# Import modules
from sklearn import tree
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted_variable
x_test = test_dataset_precictor_variables
# Create Decision Tree Regressor Object
model = tree.DecisionTreeRegressor()
# Create Decision Tree Classifier Object
model = tree.DecisionTreeClassifier()
# Train the model with training data and checking the score
model.fit(x_train, y_train)
model.score(x_train, y_train)
# Make predictions
predicted_values = model.predict(x_test)
4.朴素贝叶斯
我们再次将算法更改为 DecisionTreeRegressor:
# Import modules
from sklearn.naive_bayes import GaussianNB
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Create GaussianNB object
model = GaussianNB()
# Train the model with training data
model.fit(x_train, y_train)
# Make predictions
predicted_values = model.predict(x_test)
5.支持向量机
在本例中,我们使用 SVM 库的 SVC 类。如果是 SVR,它就是一个回归函数:
# Import modules
from sklearn import svm
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Create SVM Classifier object
model = svm.svc()
# Train the model with training data and checking the score
model.fit(x_train, y_train)
model.score(x_train, y_train)
# Make predictions
predicted_values = model.predict(x_test)
6.K- 最近邻
在 KneighborsClassifier 算法中,我们有一个超参数叫做 n_neighbors,就是我们对这个算法进行调整。
# Import modules
from sklearn.neighbors import KNeighborsClassifier
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Create KNeighbors Classifier Objects
KNeighborsClassifier(n_neighbors = 6) # default value = 5
# Train the model with training data
model.fit(x_train, y_train)
# Make predictions
predicted_values = model.predict(x_test)
7.K- 均值
# Import modules
from sklearn.cluster import KMeans
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Create KMeans objects
k_means = KMeans(n_clusters = 3, random_state = 0)
# Train the model with training data
model.fit(x_train)
# Make predictions
predicted_values = model.predict(x_test)
8.随机森林
# Import modules
from sklearn.ensemble import RandomForestClassifier
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Create Random Forest Classifier objects
model = RandomForestClassifier()
# Train the model with training data
model.fit(x_train, x_test)
# Make predictions
predicted_values = model.predict(x_test)
9.降维
# Import modules
from sklearn import decomposition
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Creating PCA decomposition object
pca = decomposition.PCA(n_components = k)
# Creating Factor analysis decomposition object
fa = decomposition.FactorAnalysis()
# Reduc the size of the training set using PCA
reduced_train = pca.fit_transform(train)
# Reduce the size of the training set using PCA
reduced_test = pca.transform(test)
10.梯度提升和 AdaBoost
# Import modules
from sklearn.ensemble import GradientBoostingClassifier
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Creating Gradient Boosting Classifier object
model = GradientBoostingClassifier(n_estimators = 100, learning_rate = 1.0, max_depth = 1, random_state = 0)
# Training the model with training data
model.fit(x_train, x_test)
# Make predictions
predicted_values = model.predict(x_test)
我们的工作将是把这些算法中的每一个块转化为一个项目。首先,定义一个业务问题,对数据进行预处理,训练算法,调整超参数,获得可验证的结果,在这个过程中不断迭代,直到我们达到满意的精度,做出理想的预测。
原文链接:
更多内容推荐
算法题每日一练 --- 第 6 天:李白打酒
话说大诗人李白,一生好饮。幸好他从不开车。
2022-07-23
80|怎样将已有算法改造成符合项目的特定算法?
2023-01-11
【使用 Python 实现算法】01 语言特性
本系列博客是我根据个人使用 Python 工作和刷题的经验总结的一些使用 Python 实现各类算法的一些技巧。
2022-06-27
文心一言 VS 讯飞星火 VS chatgpt (22)-- 算法导论 4.2 2 题
Strassen算法是一种用于矩阵乘法的算法,它的核心思想是通过不断将原始矩阵分割成较小的子矩阵来实现高效的乘法运算。以下是Strassen算法的伪代码:
2023-05-25
日拱算法:什么是“煎饼排序”?
什么是“煎饼排序”?
2022-08-30
动手实践丨基于 ModelAtrs 使用 A2C 算法制作登月器着陆小游戏
在本案例中,我们将展示如何基于A2C算法,训练一个LunarLander小游戏。
2022-11-23
vivo 故障定位平台的探索与实践
本文基于故障定位项目的实践,围绕根因定位算法的原理进行展开介绍。
2023-01-09
自定义模块:如何编写一个完整功能?
2022-12-28
79|怎样将 Python 和 C++ 结合起来混合编程?
2023-01-11
手机拍照算法和硬件哪个更重要
手机拍照算法和硬件哪个更重要?手机算法比摄像头重要
2022-04-21
GitHub 上最牛逼的 Java 教程,标星 yyds:算法
====
2022-05-09
47|小试牛刀:如何使用 Python 合并多个文件?
2022-12-07
必知必会的数学模型
2022-11-07
人工智能机器学习之 Bagging 算法
bagging(装袋算法)的集成学习方法非常简单假设我们有一个数据集,使用bootstrap sample(有放回的随机采样,每一个样本被抽中概率符合均匀分布)取了n份,作为新的训练集我们使用这n个子集分别训练一个分类器(使用分类、回归等算法)最后会得到n个分类模型。
2022-11-14
学术论坛第七期:基于统计的预测算法
学术论坛第七期:基于统计的预测算法 本次学术论坛我们邀请了云智慧算法实习生、北京科技大学硕士在读生朱同学为我们简要介绍几类轻量级的统计预测算法模型,其中包括ARMA模型、ARCH模型、HoltWinters模型、facebook提出的prophet模型以及树模型。
2022-02-18
11|字符串(下):Python 是如何处理单词的?
2022-11-16
文心一言 VS 讯飞星火 VS chatgpt (25)-- 算法导论 4.2 7 题
可以使用如下算法来计算复数 a+bi 和 c+di 的积,且只需进行三次实数乘法:
2023-05-29
算法题每日一练:矩阵置零
给定一个 m x n 的矩阵,如果一个元素为 0 ,则将其所在行和列的所有元素都设为 0 。请使用 原地算法 。
2023-05-01
文心一言 VS 讯飞星火 VS chatgpt (24)-- 算法导论 4.2 6 题
Strassen 算法是一种用于矩阵乘法的分治算法,它将原始的矩阵分解为较小的子矩阵,然后使用子矩阵相乘的结果来计算原始矩阵的乘积。
2023-05-28
2022 年顶级机器学习算法和 Python 库
这些算法之所以与众不同,是因为它们包含了一些在其它算法中并不普遍的优点。
推荐阅读
22|YouTubeDNN:召回算法的后起之秀(下)
2023-06-05
面试官:你工作了 3 年了,这道算法题你都答不出来?
2023-09-27
类人任务(human-like task)的模型
2023-12-01
大模型训练:数据与算法的关键融合
2023-10-17
CART 算法解密:从原理到 Python 实现
2023-11-24
14. 点检制的“八定”
2023-10-17
opencv 目标检测之 canny 算法
2023-06-26
电子书
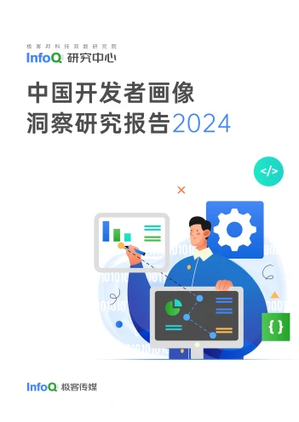
大厂实战PPT下载
换一换 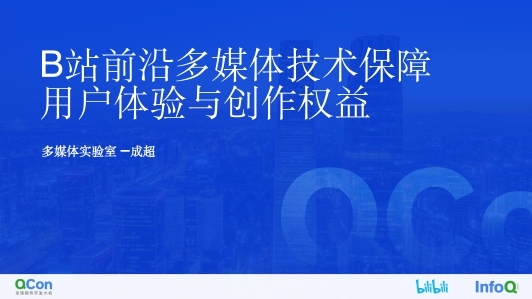
成超 | B 站 多媒体实验室算法负责人
王育军 | 小米 语音技术负责人
薛金宝 | 腾讯 机器学习平台部大模型训练框架研发技术专家
评论