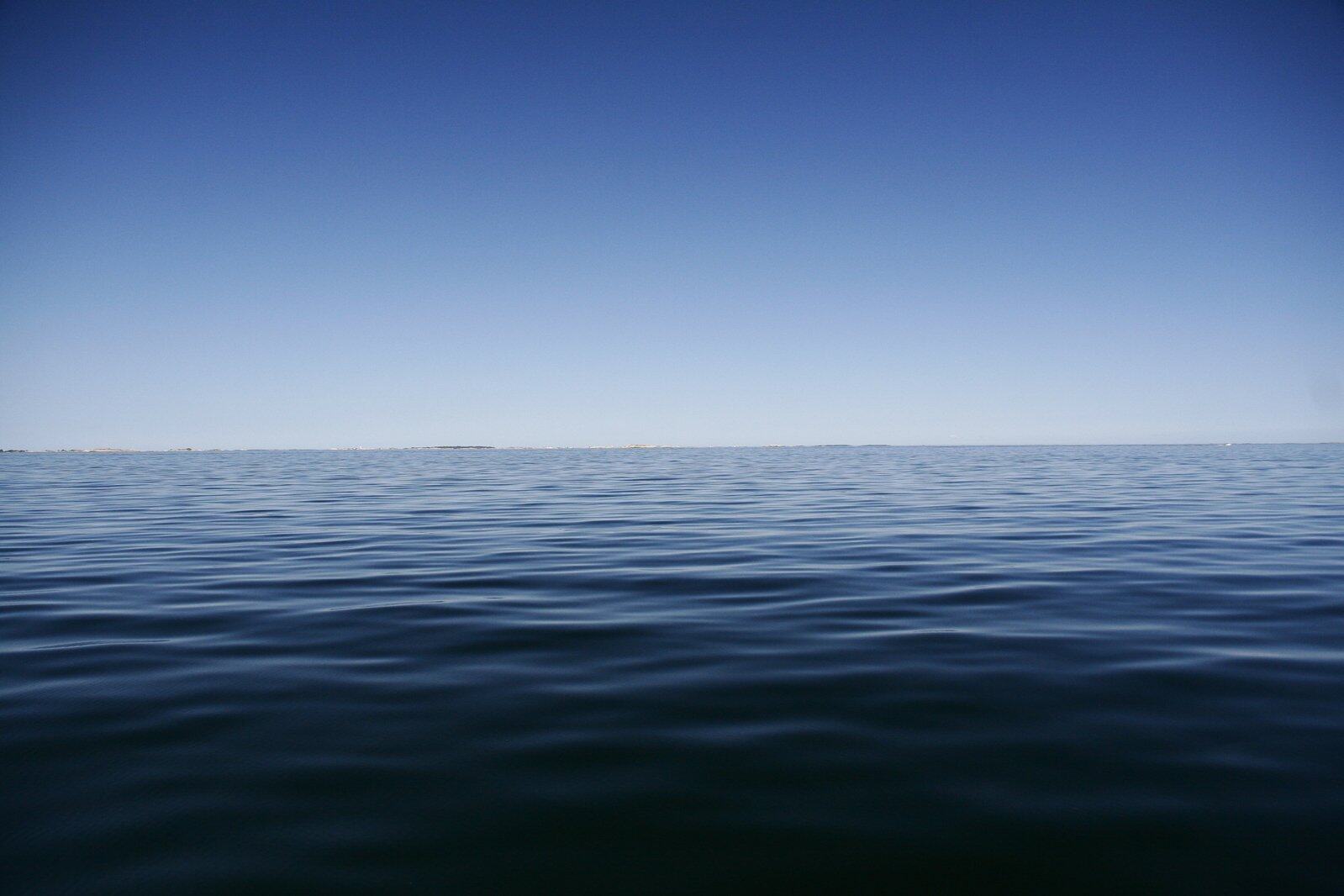
3 配置 Cognito identity pool 及 IAM 角色
3.1 创建 Identity pool 及 IAM role
3.2 配置 Developer provider name
3.3 创建 IAM 角色
利用 identity pool 生成的 身份证书管理 CMK(权限已在 CMK 管理中指定),删除其他的权限
4 实现 Cognito Developer provider
Java
/*
*
* Copyright 2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except
* in compliance with the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
*/
package com.amazon.saas.idp;
import java.util.Collections;
import java.util.Map;
import com.amazonaws.auth.DefaultAWSCredentialsProviderChain;
import com.amazonaws.services.cognitoidentity.AmazonCognitoIdentity;
import com.amazonaws.services.cognitoidentity.AmazonCognitoIdentityClientBuilder;
import com.amazonaws.services.cognitoidentity.model.GetCredentialsForIdentityRequest;
import com.amazonaws.services.cognitoidentity.model.GetCredentialsForIdentityResult;
import com.amazonaws.services.cognitoidentity.model.GetOpenIdTokenForDeveloperIdentityRequest;
import com.amazonaws.services.cognitoidentity.model.GetOpenIdTokenForDeveloperIdentityResult;
public class CognitoDeveloperIdentityProvider {
private String region = "cn-north-1";
//cognito 对于 sts 的 provider id 中国区选择 "cognito-identity.cn-north-1.amazonaws.com.cn"
private String cognitoProviderId= "cognito-identity.cn-north-1.amazonaws.com.cn"
// 前面配置的 Developer Identity Provider Name
private String developerIdentityProviderName = "developerIdentityProviderName";
// indentity pool id 如 cn-north-1:cc310c44-de78-4661-8e6e-8cc21d974058
private String identityPoolId="cn-north-1:cc310c44-de78-4661-8e6e-8cc21d974058";
private AmazonCognitoIdentity amazonCognitoIdentity;
// 构建 AmazonCognitoIdentityClient
public void init() {
AmazonCognitoIdentityClientBuilder cidBuilder = AmazonCognitoIdentityClientBuilder.standard();
cidBuilder.setRegion(region);
cidBuilder.setCredentials(DefaultAWSCredentialsProviderChain.getInstance());
amazonCognitoIdentity = cidBuilder.build();
}
//获取 cognito OpenIdToken
public GetOpenIdTokenForDeveloperIdentityResult getOpenIdTokenFromCognito(String tenantid, String userid) {
GetOpenIdTokenForDeveloperIdentityRequest request = new GetOpenIdTokenForDeveloperIdentityRequest();
request.setIdentityPoolId(identityPoolId);
Map<String, String> logins = Collections.singletonMap(developerIdentityProviderName, tenantid + ":" + userid);
request.setLogins(logins);
GetOpenIdTokenForDeveloperIdentityResult result = amazonCognitoIdentity
.getOpenIdTokenForDeveloperIdentity(request);
return result;
}
//获取 aws credentials 用于调用 KMS
public GetCredentialsForIdentityResult getCredentialsForIdentity(String identityid, String token, TenantUserInfo userinfo) {
GetCredentialsForIdentityRequest request = new GetCredentialsForIdentityRequest();
request.setIdentityId(identityid);
request.setLogins(Collections.singletonMap(cognitoProviderId, token));
GetCredentialsForIdentityResult result = amazonCognitoIdentity.getCredentialsForIdentity(request);
return result;
}
}
5 实现数据加密解密
5.1 AWS 证书与 data key 缓存
Java
/*
*
* Copyright 2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except
* in compliance with the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
*/
package com.amazon.saas.tes;
import java.util.concurrent.TimeUnit;
import com.amazon.saas.tes.TenantCryptoMaterialsManagerHolder.Cachekey;
import com.amazonaws.auth.BasicSessionCredentials;
import com.amazonaws.encryptionsdk.CryptoMaterialsManager;
import com.amazonaws.encryptionsdk.MasterKeyProvider;
import com.amazonaws.encryptionsdk.caching.CachingCryptoMaterialsManager;
import com.amazonaws.encryptionsdk.caching.CryptoMaterialsCache;
import com.amazonaws.encryptionsdk.caching.LocalCryptoMaterialsCache;
import com.amazonaws.encryptionsdk.kms.KmsMasterKey;
import com.amazonaws.encryptionsdk.kms.KmsMasterKeyProvider;
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import lombok.AllArgsConstructor;
import lombok.EqualsAndHashCode;
import lombok.Getter;
import lombok.Setter;
public class TenantCryptoMaterialsManagerHolder extends CacheLoader<Cachekey, CryptoMaterialsManager> {
@Getter
@Setter
@AllArgsConstructor
public static class Cachekey {
private String tenantID;
private String userid;
}
@Data
public static class Config {
private String keyArn;
private int maxCacheSize;
private int maxEntryAge;
private int credentialsDuration;
}
private LoadingCache<Cachekey, CryptoMaterialsManager> cache;
private Config config;
public void init() {
//配置缓存参数
config=new Config();
cache = CacheBuilder.newBuilder().maximumSize(10000)
.expireAfterWrite(config.getCredentialsDuration(), TimeUnit.MINUTES).build(this);
}
public CryptoMaterialsManager createMaterialsManager(Cachekey key) {
Credentials credentialsForIdentity = cognitoDeveloperIdentityProviderClient;
MasterKeyProvider<KmsMasterKey> keyProvider = KmsMasterKeyProvider.builder()
.withKeysForEncryption(config.getKeyArn())
.withCredentials(new BasicSessionCredentials(credentialsForIdentity.getAccessKeyId(),
credentialsForIdentity.getSecretKey(), credentialsForIdentity.getSessionToken()))
.build();
int MAX_CACHE_SIZE = config.getMaxCacheSize();
CryptoMaterialsCache cache = new LocalCryptoMaterialsCache(MAX_CACHE_SIZE);
int MAX_ENTRY_AGE_SECONDS = config.getMaxEntryAge();
int MAX_ENTRY_MSGS = config.getMaxCacheSize();
CryptoMaterialsManager cachingCmm = CachingCryptoMaterialsManager.newBuilder()
.withMasterKeyProvider(keyProvider).withCache(cache).withMaxAge(MAX_ENTRY_AGE_SECONDS, TimeUnit.SECONDS)
.withMessageUseLimit(MAX_ENTRY_MSGS).build();
return cachingCmm;
}
public CryptoMaterialsManager getMaterialsManager(TenantUserInfo userinfo) {
return cache.get(new Cachekey(userinfo.getTenantID(), userinfo.getUserid()));
}
@Override
public CryptoMaterialsManager load(Cachekey key) throws Exception {
return createMaterialsManager(key);
}
}
5.2 数据加密解密
Java
/*
*
* Copyright 2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except
* in compliance with the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
*/
package com.amazon.saas.tes;
import java.util.Collections;
import com.amazonaws.encryptionsdk.AwsCrypto;
import com.amazonaws.encryptionsdk.CryptoMaterialsManager;
import com.amazonaws.encryptionsdk.CryptoResult;
import org.springframework.beans.factory.annotation.Autowired;
public class EncDecService {
private final AwsCrypto crypto = new AwsCrypto();
@Autowired
TenantCryptoMaterialsManagerHolder tenantCryptoMaterialsManagerHolder;
public TESEncryptedMessage encrypt(String plaintext, String authorizationHeader, TenantUserInfo userinfo) {
CryptoMaterialsManager cmm = tenantCryptoMaterialsManagerHolder.getMaterialsManager(authorizationHeader,
userinfo);
String message = crypto.encryptString(cmm, plaintext,
Collections.singletonMap("tenantid.userid", userinfo.getTenantID() + "." + userinfo.getUserid()))
.getResult();
TESEncryptedMessage re = new TESEncryptedMessage();
re.setEncyptedMsg(message);
return re;
}
public TesPlainText dectypt(String encryptMessage, String authorizationHeader, TenantUserInfo userinfo) {
CryptoMaterialsManager cmm = tenantCryptoMaterialsManagerHolder.getMaterialsManager(authorizationHeader,
userinfo);
CryptoResult<String, ?> decryptResult = crypto.decryptString(cmm, encryptMessage);
TesPlainText re = new TesPlainText();
re.setText(decryptResult.getResult());
return re;
}
作者介绍:
*
### [](https://amazonaws-china.com/cn/blogs/china/tag/%E4%BB%BB%E8%80%80%E6%B4%B2/)
AWS解决方案架构师,负责企业客户应用在AWS的架构咨询和 设计。在微服务架构设计、数据库等领域有丰富的经验
本文转载自 AWS 技术博客。
原文链接:https://amazonaws-china.com/cn/blogs/china/aws-kms-enables-secure-data-encryption-across-tenants/
更多内容推荐
19|自托管构建:如何使用 Harbor 搭建企业级镜像仓库?
这节课,我们来学习如何使用 Harbor 来搭建企业级的镜像仓库。
2023-01-20
Java 中 restTemplate 的使用
原文链接
2023-01-13
2022 年 Java 行业分析报告
前段时间介绍了从 Java8 到 Java17 每个版本比较有特点的新特性(收录在《从小工到专家的 Java 进阶之旅》专栏),今天看到 JRebel 发布了《2022 年 Java 发展趋势和分析》,于是借此分析一下 Java 行业的现状,希望给大家一些参考。
2022-06-19
Java 中的常量和变量
常量:是指在Java程序中固定不变的数据。
2022-10-10
18|自托管构建:如何使用 Tekton 构建镜像?
这节课,我们来介绍其中一种自动构建镜像的自托管方案:使用 Tekton 来自动构建镜像。
2023-01-18
32|存储引擎:数据清洗与存储
这节课,我们一起写一个存储引擎,用它来处理数据的存储问题。
2022-12-22
17|组件监控:Kubernetes Node 组件的关键指标与数据采集
Kubernetes Node组件的关键指标与数据采集
2023-02-15
Java 中 Get 和 Post 的使用
原文链接
2023-01-12
如何写好一个 Java 类?
在进行程序开发的过程中,我们有时会看到这样的Java类:
2022-02-28
09|如何迁移应用配置?
这节课,我们来看看 K8s 应用读取配置信息的最佳实践。
2022-12-28
java 零基础入门 -Java 反射机制
哈喽,各位小伙伴们好,我是喵手。day3
2022-07-08
爆火!阿里新版 23 年面试突击进阶手册,Github 标星 51k!
目前Java后端就业前景怎么样?,Java就业大环境仍然根基稳定,市场上有很多机会,技术好的人前景就好,就看你有多大本事了。或者换句话,Java是市场上需求量最大的岗位,如果Java不行,那其他的就行么?
2023-03-18
Java 开发基础之开发环境搭建
Java开发基础之开发环境搭建
2021-11-25
8000 字详解 Thread Pool Executor
Java是如何实现和管理线程池的?
2022-12-21
15|mBatis:如何将 SQL 语句配置化?
mBatis:如何将SQL语句配置化?
2023-04-14
Java 设计模式学习总结
设计模式的三大类
2022-06-06
Java 中 restTemplate 携带 Header 请求
创建请求头:
2023-03-09
Java 性能优化的 35 个细节(珍藏版)
例如:
2022-04-16
推荐阅读
Java 服务总在半夜挂,背后的真相竟然是... | 京东云技术团队
2023-10-25
23|数据库应用(三):项目数据库配置实战
2023-06-14
优化 Java 代码效率和算法设计,提升性能
2023-09-19
加餐|GPT 编程(上) :如何用 ChatGPT 辅助我们编程?
2023-05-15
13.Registry
2023-09-30
Java 实现多用户即时通信系统
2023-05-10
后端除了增删改查还有什么?
2023-11-16
电子书
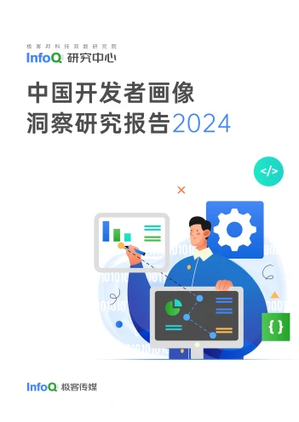
大厂实战PPT下载
换一换 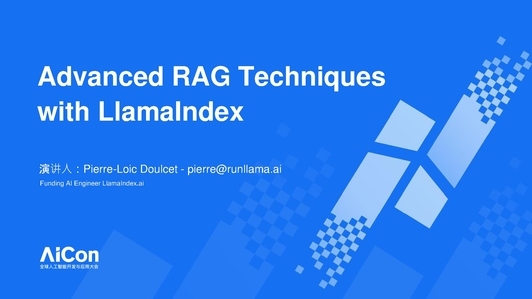
Pierre-Loic Doulcet | LlamaIndex 创始 AI 工程师
陈孟斌 | 前滴滴两轮车 产品技术负责人
郭东白 | Coupang 副总裁
评论