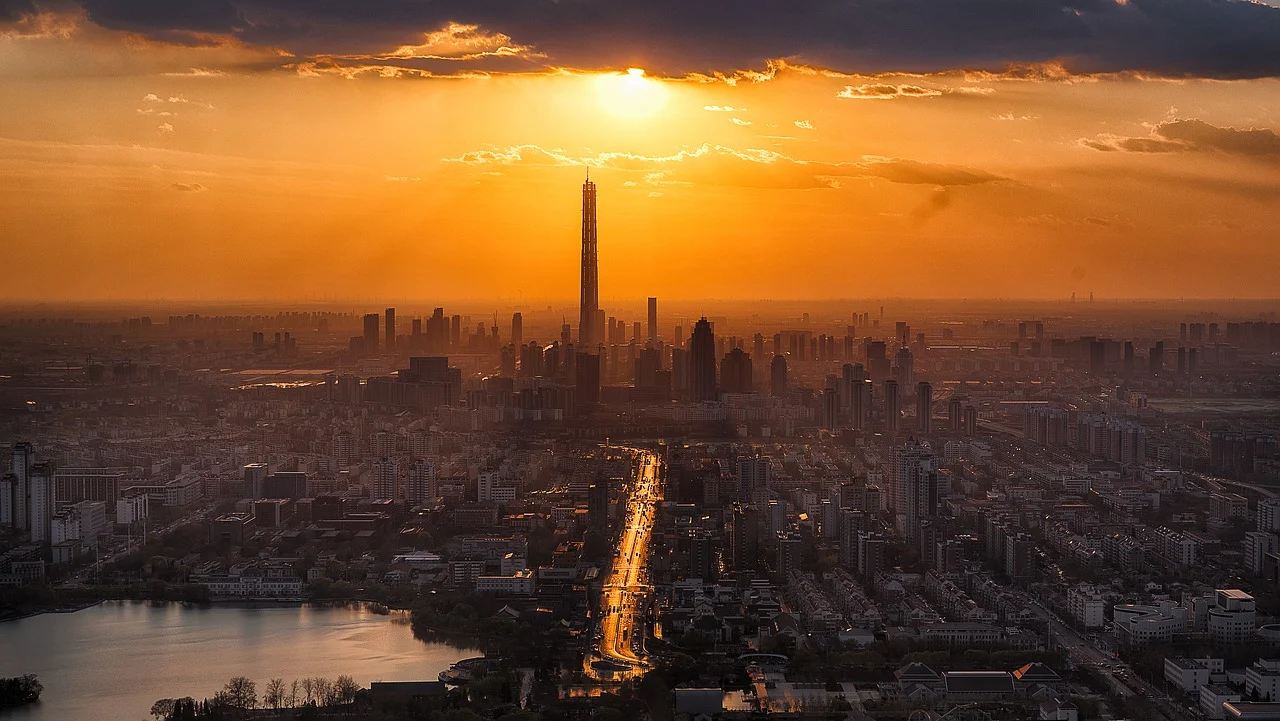
本文,我们将通过 Python 语言包,来构建一些机器学习模型。
构建机器学习模型的模板
该 Notebook 包含了用于创建主要机器学习算法所需的代码模板。在 scikit-learn 中,我们已经准备好了几个算法。只需调整参数,给它们输入数据,进行训练,生成模型,最后进行预测。
1.线性回归
对于线性回归,我们需要从 sklearn 库中导入 linear_model。我们准备好训练和测试数据,然后将预测模型实例化为一个名为线性回归 LinearRegression 算法的对象,它是 linear_model 包的一个类,从而创建预测模型。之后我们利用拟合函数对算法进行训练,并利用得分来评估模型。最后,我们将系数打印出来,用模型进行新的预测。
# Import modules
from sklearn import linear_model
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted_variable
x_test = test_dataset_precictor_variables
# Create linear regression object
linear = linear_model.LinearRegression()
# Train the model with training data and check the score
linear.fit(x_train, y_train)
linear.score(x_train, y_train)
# Collect coefficients
print('Coefficient: \n', linear.coef_)
print('Intercept: \n', linear.intercept_)
# Make predictions
predicted_values = linear.predict(x_test)
2.逻辑回归
在本例中,从线性回归到逻辑回归唯一改变的是我们要使用的算法。我们将 LinearRegression 改为 LogisticRegression。
# Import modules
from sklearn.linear_model import LogisticRegression
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted_variable
x_test = test_dataset_precictor_variables
# Create logistic regression object
model = LogisticRegression()
# Train the model with training data and checking the score
model.fit(x_train, y_train)
model.score(x_train, y_train)
# Collect coefficients
print('Coefficient: \n', model.coef_)
print('Intercept: \n', model.intercept_)
# Make predictions
predicted_vaues = model.predict(x_teste)
3.决策树
我们再次将算法更改为 DecisionTreeRegressor:
# Import modules
from sklearn import tree
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted_variable
x_test = test_dataset_precictor_variables
# Create Decision Tree Regressor Object
model = tree.DecisionTreeRegressor()
# Create Decision Tree Classifier Object
model = tree.DecisionTreeClassifier()
# Train the model with training data and checking the score
model.fit(x_train, y_train)
model.score(x_train, y_train)
# Make predictions
predicted_values = model.predict(x_test)
4.朴素贝叶斯
我们再次将算法更改为 DecisionTreeRegressor:
# Import modules
from sklearn.naive_bayes import GaussianNB
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Create GaussianNB object
model = GaussianNB()
# Train the model with training data
model.fit(x_train, y_train)
# Make predictions
predicted_values = model.predict(x_test)
5.支持向量机
在本例中,我们使用 SVM 库的 SVC 类。如果是 SVR,它就是一个回归函数:
# Import modules
from sklearn import svm
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Create SVM Classifier object
model = svm.svc()
# Train the model with training data and checking the score
model.fit(x_train, y_train)
model.score(x_train, y_train)
# Make predictions
predicted_values = model.predict(x_test)
6.K- 最近邻
在 KneighborsClassifier 算法中,我们有一个超参数叫做 n_neighbors,就是我们对这个算法进行调整。
# Import modules
from sklearn.neighbors import KNeighborsClassifier
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Create KNeighbors Classifier Objects
KNeighborsClassifier(n_neighbors = 6) # default value = 5
# Train the model with training data
model.fit(x_train, y_train)
# Make predictions
predicted_values = model.predict(x_test)
7.K- 均值
# Import modules
from sklearn.cluster import KMeans
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Create KMeans objects
k_means = KMeans(n_clusters = 3, random_state = 0)
# Train the model with training data
model.fit(x_train)
# Make predictions
predicted_values = model.predict(x_test)
8.随机森林
# Import modules
from sklearn.ensemble import RandomForestClassifier
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Create Random Forest Classifier objects
model = RandomForestClassifier()
# Train the model with training data
model.fit(x_train, x_test)
# Make predictions
predicted_values = model.predict(x_test)
9.降维
# Import modules
from sklearn import decomposition
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Creating PCA decomposition object
pca = decomposition.PCA(n_components = k)
# Creating Factor analysis decomposition object
fa = decomposition.FactorAnalysis()
# Reduc the size of the training set using PCA
reduced_train = pca.fit_transform(train)
# Reduce the size of the training set using PCA
reduced_test = pca.transform(test)
10.梯度提升和 AdaBoost
# Import modules
from sklearn.ensemble import GradientBoostingClassifier
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Creating Gradient Boosting Classifier object
model = GradientBoostingClassifier(n_estimators = 100, learning_rate = 1.0, max_depth = 1, random_state = 0)
# Training the model with training data
model.fit(x_train, x_test)
# Make predictions
predicted_values = model.predict(x_test)
我们的工作将是把这些算法中的每一个块转化为一个项目。首先,定义一个业务问题,对数据进行预处理,训练算法,调整超参数,获得可验证的结果,在这个过程中不断迭代,直到我们达到满意的精度,做出理想的预测。
原文链接:
更多内容推荐
算法题每日一练: 青蛙跳台阶
一只青蛙一次可以跳上1级台阶,也可以跳上2级台阶。求该青蛙跳上一个 n (0 <= n <= 100)级的台阶总共有多少种跳法。
2023-04-26
2022 年顶级机器学习算法和 Python 库
这些算法之所以与众不同,是因为它们包含了一些在其它算法中并不普遍的优点。
MoBYv2AL :结合 BOYL 和 MoCo 的主动学习算法
MoBYv2AL 将自监督应用到主动学习
2023-02-03
Python 代码阅读(第 76 篇):摄氏温度与华氏温度互转
本篇阅读的代码实现了摄氏温度与华氏温度的相互转换。 本篇阅读的代码片段来自于30-seconds-of-python。
2022-02-25
04|新时代模型性能大比拼,GPT-3 到底胜在哪里?
这一讲我们一起使用 Fasttext、T5-small 和 T5-base 这三个预训练模型,做零样本分类测试。
2023-03-27
拯救你的算法!GitHub 上神仙项目手把手带你刷算法,Star 数已破 110k
前不久在 GitHub 出现了一个手把手带你刷 算法的项目:fucking-algorithm。该项目此前在 GitHub 开源后,连续多次霸榜 GitHub Trending 首页,用了一周Star数便突破 110k,受欢迎程度由此可见一斑:
2021-09-14
大厂算法面试之 leetcode 精讲 12. 堆
1.开篇介绍
2021-11-30
如何在 Django 中创建应用程序?
先决条件——如何在 Django 中使用 MVT 创建基本项目?
2022-06-15
顶会 VLDB‘22 论文解读:CAE-ENSEMBLE 算法
摘要:针对时间序列离群点检测问题,提出了基于CNN-AutoEncoder和集成学习的CAE-ENSEMBLE深度神经网络算法,并通过大量的实验证明CAE-ENSEMBLE算法能有效提高时间序列离群点检测的准确度与效率。
2021-11-04
17|集成学习:机器学习模型如何“博采众长”?
集成学习在机器学习中是很特别的一类方法,能够处理回归和分类问题,而且它对于避免模型中的过拟合问题,具有天然的优势。
2021-10-06
05|善用 Embedding,我们来给文本分分类
这一讲你会学到如何利用OpenAI来获取文本的Embedding,然后通过传统的机器学习方式进行训练,并评估训练的结果。
2023-03-28
Python 新手太需要了,这 5 个做题练习网站爱了!
程序员宝藏库:https://gitee.com/sharetech_lee/CS-Books-Store
2023-02-04
24|GBDT+LR:排序算法经典中的经典
在前面的课程中,我们讲了推荐系统中的数据处理、接口实现和一些召回算法和模型,从本章开始,我们就会进入一个新的篇章:推荐系统中的排序算法。
2023-06-09
7 大特征交互模型,最好的深度学习推荐算法总结
深度学习自出现以来,不断改变着人工智能领域的技术发展,推荐系统领域的研究同样也受到了深远的影响。
2022-08-19
什么是 Spring-Cloud、需要掌握哪些知识点,Java 面试常问的算法题
zuul:routes:consumer1: /FrancisQ1/**consumer2: /FrancisQ2/**
2021-09-11
23|OpenClip:让我们搞清楚图片说了些什么
OpenClip:让我们搞清楚图片说了些什么?
2023-04-28
前端之算法(九)回溯算法
大家好,今天我们要聊的是回溯算法这种方法,它和贪心算法、分而治之、动态规划一样,也是算法设计中的一种方法,你同样可以把它当作解决问题的一种思路。接下来让我来看看回溯算法是什么?
2021-08-19
80|怎样将已有算法改造成符合项目的特定算法?
2023-01-11
大厂算法面试之 leetcode 精讲 13. 单调栈
1.开篇介绍
2021-12-01
推荐阅读
华为云耀云服务器 L 实例:中小企业数字化转型的智选
2023-11-26
25|模型工程(一):如何让你的训练数据无中生有?
2023-10-16
14|实战:通过 RFM 值给用户画像
2023-11-27
大模型训练:数据与算法的关键融合
2023-10-17
计算网络之 MSTP 协议与 VRRP 协议
2023-11-16
使用 Python 调用 API 接口获取淘宝商品数据
2023-11-13
8. Spark 实战案例(二)
2023-09-08
电子书
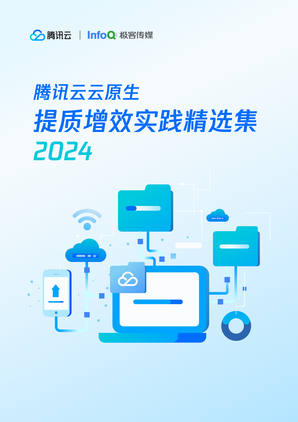
大厂实战PPT下载
换一换 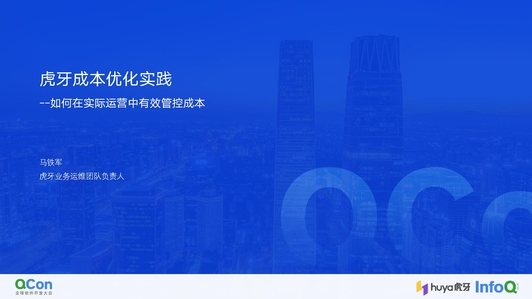
马铁军 | 虎牙 业务运维负责人
徐瑶 | Fabarta 向量引擎研发专家、Apache Kudu PMC Member
郑红波 | 星图金融 研发中心测试管理部总监
评论