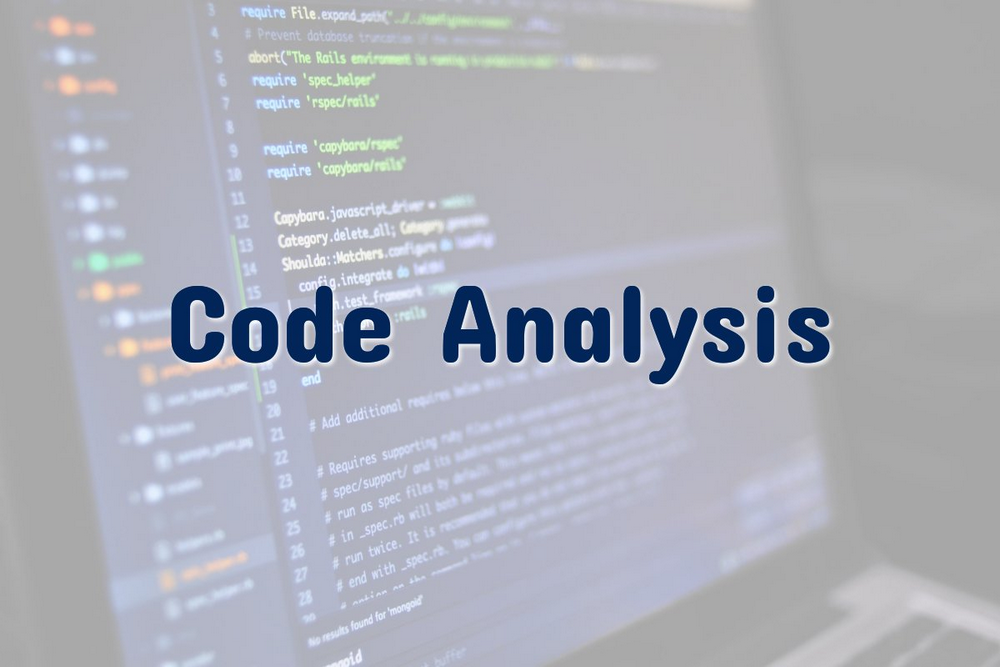
详细分析 ClassLoader 加载原理
ClassLoader 的继承关系如下:
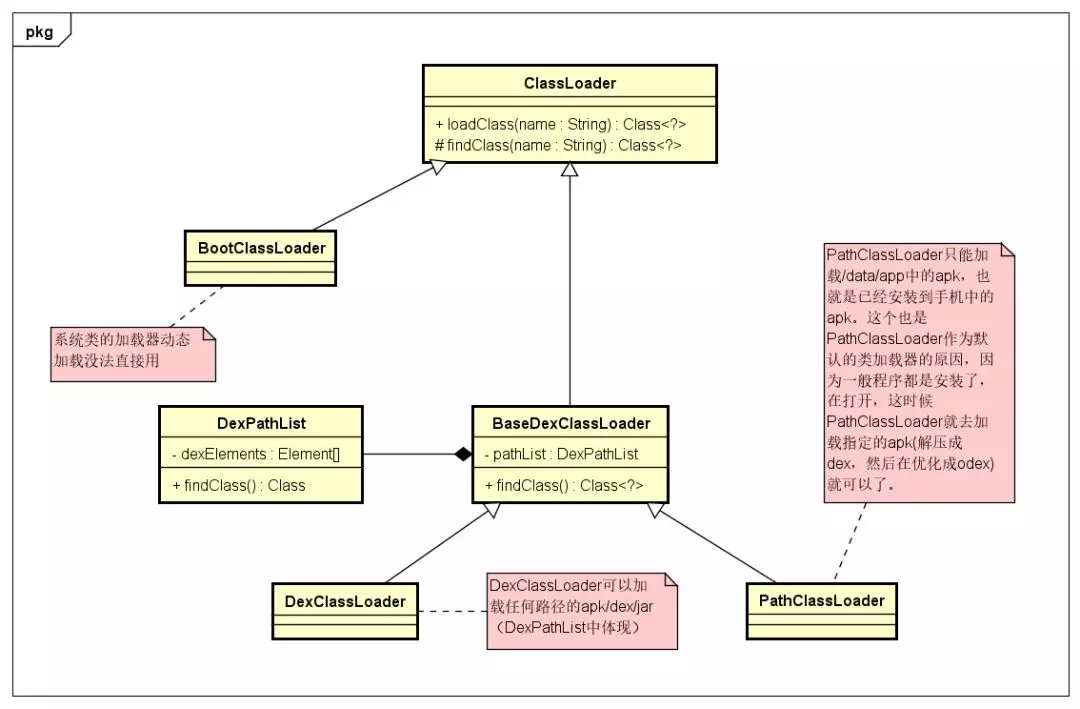
这里我们主要分析一下 BaseDexClassLoader.findClass()
和 ClassLoader.loadClass()
两个函数在系统中是怎么进行查找 class 的过程。
我们看一下系统加载类ClassLoader.loadClass()
函数实现代码,在ClassLoader.java
中:
protected Class<?> loadClass(String name, boolean resolve)
throws ClassNotFoundException
{
// 首先 检测是否已经加载过
Class<?> c = findLoadedClass(name);
if (c == null) {
try {
if (parent != null) {
//去调用父类的loadClass
c = parent.loadClass(name, false);
} else {
c = findBootstrapClassOrNull(name);
}
} catch (ClassNotFoundException e) {
// ClassNotFoundException thrown if class not found
// from the non-null parent class loader
}
if (c == null) {
//未找到的情况下,使用findClass在当前dex查找
c = findClass(name);
}
}
return c;
}
protected Class<?> findClass(String name) throws ClassNotFoundException {
throw new ClassNotFoundException(name);
}
1,
loadClass()
先调用findLoadedClass()
来判断当前类是否已加载;2, 未查找到递归去父类中查找是否加载到缓存;
3, 均未缓存,去
BootClassLoader
中查找;4, 以上未发现,自顶级父类依次向下查找,调用
findClass()
查找当前 dex。
findLoadedClass 函数分析
下图为
findLoadedClass()
的调用流程;根据调用流程图配合源代码进行详细的分析原理。
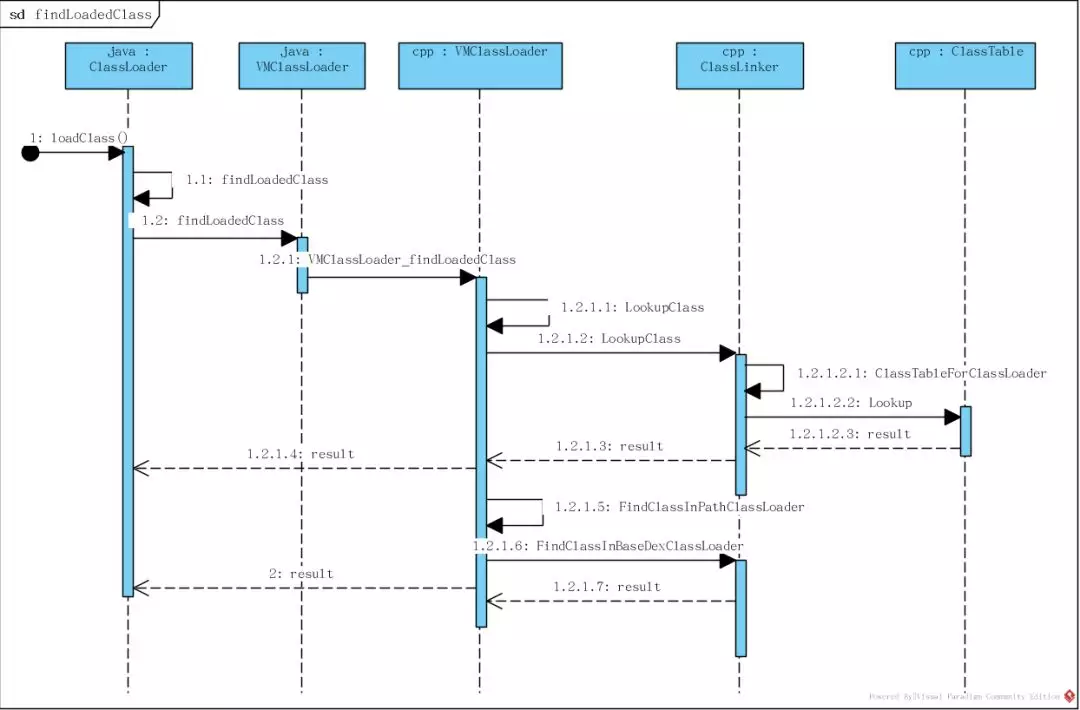
下面介绍对应的源代码实现部分:
protected final Class<?> findLoadedClass(String name) {
ClassLoader loader;
if (this == BootClassLoader.getInstance())
loader = null;
else
loader = this;
return VMClassLoader.findLoadedClass(loader, name);
}
函数最终统一调用VMClassLoader.findLoadedClass()
进行查找类。
native static Class findLoadedClass(ClassLoader cl, String name);
实现在java_lang_VMClassLoader.cc
文件中。
static jclass VMClassLoader_findLoadedClass(JNIEnv* env, jclass, jobject javaLoader,jstring javaName) {
....
ObjPtr<mirror::ClassLoader> loader = soa.Decode<mirror::ClassLoader>(javaLoader);
ClassLinker* cl = Runtime::Current()->GetClassLinker();
ObjPtr<mirror::Class> c = VMClassLoader::LookupClass(cl,
soa.Self(),
descriptor.c_str(),
descriptor_hash,
loader);
if (c != nullptr && c->IsResolved()) {
return soa.AddLocalReference<jclass>(c);
}
...
if (loader != nullptr) {
// Try the common case.
StackHandleScope<1> hs(soa.Self());
c = VMClassLoader::FindClassInPathClassLoader(cl,
soa,
soa.Self(),
descriptor.c_str(),
descriptor_hash,
hs.NewHandle(loader));
if (c != nullptr) {
return soa.AddLocalReference<jclass>(c);
}
}
return nullptr;
}
static mirror::Class* LookupClass(ClassLinker* cl,
Thread* self,
const char* descriptor,
size_t hash,
ObjPtr<mirror::ClassLoader> class_loader)
REQUIRES(!Locks::classlinker_classes_lock_)
REQUIRES_SHARED(Locks::mutator_lock_) {
return cl->LookupClass(self, descriptor, hash, class_loader);
}
static ObjPtr<mirror::Class> FindClassInPathClassLoader(ClassLinker* cl,
ScopedObjectAccessAlreadyRunnable& soa,
Thread* self,
const char* descriptor,
size_t hash,
Handle<mirror::ClassLoader> class_loader)
REQUIRES_SHARED(Locks::mutator_lock_) {
ObjPtr<mirror::Class> result;
if (cl->FindClassInBaseDexClassLoader(soa, self, descriptor, hash, class_loader, &result)) {
return result;
}
return nullptr;
}
上述代码findLoadedClass()
分为两步;
1,通过
class_linker_->Lookupclass()
进行查找加载类;2,如果没找到再通过
class_linker_->FindClassInPathClassLoader()
进行查找。
class_linker_
在虚拟机的启动startVM()
函数的时候进行的初始化。
Runtime::class_linker_
在Runtime::Init()
函数的时候做的初始化。
if (UNLIKELY(IsAotCompiler())) {
class_linker_ = new AotClassLinker(intern_table_);
} else {
class_linker_ = new ClassLinker(intern_table_);
}
继续来分析ClassLinker::LookupClass()
函数的具体实现:
mirror::Class* ClassLinker::LookupClass(Thread* self,
const char* descriptor,
size_t hash,
ObjPtr<mirror::ClassLoader> class_loader) {
ReaderMutexLock mu(self, *Locks::classlinker_classes_lock_);
ClassTable* const class_table = ClassTableForClassLoader(class_loader);
if (class_table != nullptr) {
ObjPtr<mirror::Class> result = class_table->Lookup(descriptor, hash);
if (result != nullptr) {
return result.Ptr();
}
}
return nullptr;
}
LookupClass()
函数通过class_loader
是否为nullptr
,nullptr
使用boot_class_table_
来获取class_table
, 否则获取当前ClassLoader
的ClassTable
。 class_table
存放当前已经加载过的 class,其实可以理解为 class cache。如何进行 dex 解析和 aot 等加载系统类和解析映射到内存中的不在此处展开分析。可以了解 art 虚拟机启动进行详细分析。
findClass()函数分析
下图是 findClass 的调用流程;根据调用流程图配合下面的代码进行详细的分析了解:
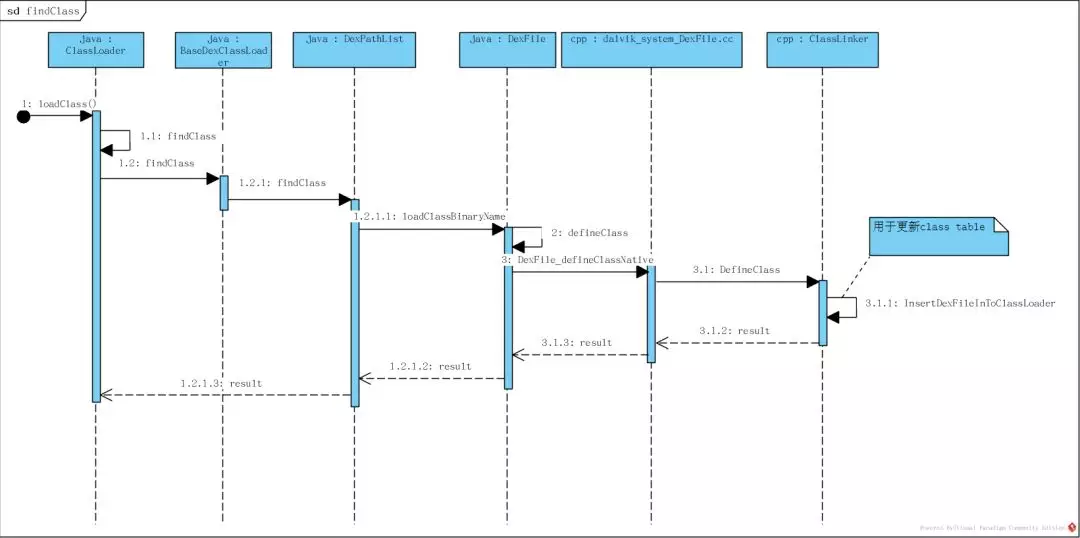
下面我们介绍对应的源代码实现部分。
findClass()
函数在BaseDexClassLoader.java
实现, 该函数主要做的事情就是在当前 dex 中查找类。如果类在当前 dex 中即返回。
代码如下:
@Override
protected Class<?> findClass(String name) throws ClassNotFoundException {
List<Throwable> suppressedExceptions = new ArrayList<Throwable>();
Class c = pathList.findClass(name, suppressedExceptions);
if (c == null) {
...
throw cnfe;
}
return c;
}
pathList
类型为DexPathList
用来保存dexfile
文件的句柄等 dex 的操作。pathList.findClass()
实现在当前 dex 中查找类, pathList
在new DexClassLoader()
构造时初始化。
public BaseDexClassLoader(String dexPath, File optimizedDirectory,
String librarySearchPath, ClassLoader parent) {
...
this.pathList = new DexPathList(this, dexPath, librarySearchPath, null);
...
}
DexPathList.java
public DexPathList(ClassLoader definingContext, String dexPath,
String librarySearchPath, File optimizedDirectory) {
...
this.definingContext = definingContext;
ArrayList<IOException> suppressedExceptions = new ArrayList<IOException>();
// save dexPath for BaseDexClassLoader
this.dexElements = makeDexElements(splitDexPath(dexPath), optimizedDirectory,
suppressedExceptions, definingContext);
this.nativeLibraryDirectories = splitPaths(librarySearchPath, false);
this.systemNativeLibraryDirectories =
splitPaths(System.getProperty("java.library.path"), true);
List<File> allNativeLibraryDirectories = new ArrayList<>(nativeLibraryDirectories);
allNativeLibraryDirectories.addAll(systemNativeLibraryDirectories);
this.nativeLibraryPathElements = makePathElements(allNativeLibraryDirectories);
if (suppressedExceptions.size() > 0) {
this.dexElementsSuppressedExceptions =
suppressedExceptions.toArray(new IOException[suppressedExceptions.size()]);
} else {
dexElementsSuppressedExceptions = null;
}
}
dexElements
数组保存 dexfile 文件句柄。具体实现在makeDexElements()
函数中调用loadDexFile()
函数加载 dex。该函数实现:
DexFile.java
private static DexFile loadDexFile(File file, File optimizedDirectory, ClassLoader loader, Element[] elements) throws IOException {
if (optimizedDirectory == null) {
return new DexFile(file, loader, elements);
} else {
String optimizedPath = optimizedPathFor(file, optimizedDirectory);
return DexFile.loadDex(file.getPath(), optimizedPath, 0, loader, elements);
}
}
DexFile.loadDex()
进行解析加载 dex 文件。关键代码如下:
private DexFile(String sourceName, String outputName, int flags, ClassLoader loader, DexPathList.Element[] elements) throws IOException {
...
mCookie = openDexFile(sourceName, outputName, flags, loader, elements);
mInternalCookie = mCookie;
mFileName = sourceName;
...
}
private static Object openDexFile(String sourceName, String outputName, int flags, ClassLoader loader, DexPathList.Element[] elements) throws IOException {
// Use absolute paths to enable the use of relative paths when testing on host.
return openDexFileNative(new File(sourceName).getAbsolutePath(),
(outputName == null)
? null
: new File(outputName).getAbsolutePath(),
flags,loader,elements);
}
private static native Object openDexFileNative(String sourceName, String outputName, int flags, ClassLoader loader, DexPathList.Element[] elements);
最终打开dexfile
是通过native
方法实现,并且返回mCookie
, mCookie
类型是int
用来标识dex
的唯一性。 openDexFileNative()
实现代码:
//`dalvik_system_DexFile.cc`
static jobject DexFile_openDexFileNative(JNIEnv* env,
jclass,
jstring javaSourceName,
jstring javaOutputName,
jint flags ATTRIBUTE_UNUSED,
jobject class_loader,
jobjectArray dex_elements)
{
...
Runtime* const runtime = Runtime::Current();
ClassLinker* linker = runtime->GetClassLinker();
...
dex_files = runtime->GetOatFileManager().OpenDexFilesFromOat(sourceName.c_str(), class_loader, dex_elements, /*out*/ &oat_file, /*out*/ &error_msgs);
....
}
上述代码通过aotManager
打开并返回mCookie
,进一步的打开实现不在此处展开。即上述已经填充elements[]
,下面开始展开pathList.findClass()
函数的查找方式。
//BaseDexClassLoader.java
public Class<?> findClass(String name, List<Throwable> suppressed) {
for (Element element : dexElements) {
Class<?> clazz = element.findClass(name, definingContext, suppressed);
if (clazz != null) {
return clazz;
}
}
if (dexElementsSuppressedExceptions != null) {
suppressed.addAll(Arrays.asList(dexElementsSuppressedExceptions));
}
return null;
}
findClass()
会遍历elements[]
, 每个element
保存了 dex 的DexFile
句柄,然后调用loadClassBinaryName()
函数进行当前 dex 查找类。
//DexPathList.java
public Class<?> findClass(String name, ClassLoader definingContext,
List<Throwable> suppressed) {
return dexFile != null ? dexFile.loadClassBinaryName(name, definingContext, suppressed): null;
}
public Class loadClassBinaryName(String name, ClassLoader loader, List<Throwable> suppressed) {
return defineClass(name, loader, mCookie, this, suppressed);
}
private static Class defineClass(String name, ClassLoader loader, Object cookie, DexFile dexFile, List<Throwable> suppressed) {
Class result = null;
try {
result = defineClassNative(name, loader, cookie, dexFile);
} catch (NoClassDefFoundError e) {
if (suppressed != null) {
suppressed.add(e);
}
} catch (ClassNotFoundException e) {
if (suppressed != null) {
suppressed.add(e);
}
}
return result;
}
真正去 dex 或者内存中查找类的函数在native
中defineClassNative()
实现, 我们来分析一下真正的实现过程:
private static native Class defineClassNative(String name, ClassLoader loader, Object cookie, DexFile dexFile)
//dalvik_system_DexFile.cc
static jclass DexFile_defineClassNative(JNIEnv* env,
jclass,
jstring javaName,
jobject javaLoader,
jobject cookie,
jobject dexFile) {
std::vector<const DexFile*> dex_files;
const OatFile* oat_file;
if (!ConvertJavaArrayToDexFiles(env, cookie, /*out*/ dex_files, /*out*/ oat_file)) {
...
return nullptr;
}
ScopedUtfChars class_name(env, javaName);
...
const std::string descriptor(DotToDescriptor(class_name.c_str()));
const size_t hash(ComputeModifiedUtf8Hash(descriptor.c_str()));
for (auto& dex_file : dex_files) {
...
ClassLinker* class_linker = Runtime::Current()->GetClassLinker();
ObjPtr<mirror::Class> result = class_linker->DefineClass(soa.Self(),
descriptor.c_str(),
hash,
class_loader,
*dex_file,
*dex_class_def);
// Add the used dex file. This only required for the DexFile.loadClass API since normal
// class loaders already keep their dex files live.
class_linker->InsertDexFileInToClassLoader(soa.Decode<mirror::Object>(dexFile),
class_loader.Get());
....
return soa.AddLocalReference<jclass>(result);
}
}
...
return nullptr;
}
通过Runtime
拿到当前的ClassLinker
对象,然后通过class_linker->DefineClass()
在当前 dex 中进行查找类。然后把找到的类通过class_linker->InsertDexFileInToClassLoader()
插入到 class_table 中进行缓存,返回查找到的类。这里不进一步展开分析。
Android ClassLoader 加载过程的源代码分析到此已经分析得差不多了,如果想深入地了解具体原理,可以自己看源代码的实现。本文就介绍到这里。初次写技术分享的文章,如有错误请指正,感谢!
本文转载自公众号 360 技术(ID:qihoo_tech)。
原文链接:
https://mp.weixin.qq.com/s/31MGkrTTjiHfXffUDydONg
更多内容推荐
SpringBoot 进阶 (叁):Spring Boot 启动过程分析
本文的分析基于Spring Boot 2.1.*,非Spring的代码只有下面这个启动main函数:
2022-08-17
cstdio 的源码学习分析 10- 格式化输入输出函数 fprintf--- 宏定义 / 辅助函数分析 06
fprintf函数的实现vfprintf中包含了相当多的宏定义和辅助函数,接下来我们一起来分析一下它们对应的源码实现。
2022-10-18
七、HikariConfig 初始化分析
HikariCP一直以高效著称,但是从来没有去研究过为什么会比其他的数据库连接池高效。后来为了排查一个数据库连接池的问题,就深入了解了一下HikariCP的源代码,然后就有了这个深入浅出的源码解析系列,不仅解释是什么,还让你知道为什么。
2022-07-29
cstdio 的源码学习分析 10- 格式化输入输出函数 fprintf--- 宏定义 / 辅助函数分析 04
fprintf函数的实现vfprintf中包含了相当多的宏定义和辅助函数,接下来我们一起来分析一下它们对应的源码实现。
2022-10-16
28|追本溯源:从第一版 React Native 开始学会读懂源码
今天,我们以第一版React Native源码为例,教你如何通过读源码,一步一步理解React Native新架构。
2022-09-01
Spring 源码解析 (十二)Spring 扩展接口 SmartInstantiationAwareBeanPostProcessor 解析
之前我们分析了 InstantiationAwareBeanPostProcessor、BeanPostProcessor、今天来分析一下SmartInstantiationAwareBeanPostProcessor的用法; SmartInstantiationAwareBeanPostProcessor 继承自 InstantiationAwareBeanPostProcessor;
2022-09-08
九、HikariCP 源码分析之 ConcurrentBag 二
HikariCP一直以高效著称,但是从来没有去研究过为什么会比其他的数据库连接池高效。后来为了排查一个数据库连接池的问题,就深入了解了一下HikariCP的源代码,然后就有了这个深入浅出的源码解析系列,不仅解释是什么,还让你知道为什么。
2022-07-29
14. Middleware:Trace 简介和 OpenTelemetry
2023-09-26
C++ 学习 ---cstdio 的源码学习分析 07- 刷新文件流函数 fflush
stdio.h中定义了一系列文件访问函数(fopen,fclose,fflush,freopen,setbuf,setvbuf),接下来我们一起来分析一下fflush对应的源码实现。
2022-10-07
利用 AndroidNativeEmu 完成多层 jni 调用的模拟
利用AndroidNativeEmu完成多层jni调用的模拟
2022-09-14
29|弄清现状:新架构预览版究竟长什么样?
今天我们的目标就是承接上一节课讲过的方法,画一张新架构的“鸟瞰图”,看看当前0.70 版本的新架构预览版究竟是怎么设计的。
2022-10-01
自主创新、领先一代,星环科技成功登陆科创板
10月18日,“国产大数据基础软件”星环信息科技(上海)股份有限公司(简称“星环科技”,证券代码“688031”)在科创板上市,宣告正式开启新征程。
2022-10-21
C++ 学习 ---cstdio 的源码学习分析 04- 创建临时文件函数 tmpfile
stdio.h中定义了文件删除函数remove,文件重命名函数rename,创建临时文件函数tmpfile,生成临时文件名函数tmpnam。接下来我们一起来分析一下tmpfile对应的源码实现。
2022-09-20
39|源码解读:V8 执行 JS 代码的全过程
这节课我会从源码理解讲起,带你了解了V8 执行 JS 代码的全过程。
2022-10-31
C++ 学习 ---cstdio 的源码学习分析 03- 文件重命名函数 rename
stdio.h中定义了文件删除函数remove,文件重命名函数rename,打开临时文件函数tmpfile,生成临时文件名函数tmpnam。接下来我们一起来分析一下rename对应的源码实现。
2022-09-18
android 图片加载库 Glide4 使用教程(项目中如何快速将 Glide3 替换成 Glide4)
获取gif第一帧
2021-11-07
C++ 学习 ---cstdio 的源码学习分析 05- 打开文件函数 fopen
stdio.h中定义了一系列文件访问函数(fopen,fclose,fflush,freopen,setbuf,setvbuf),接下来我们一起来分析一下fopen对应的源码实现。
2022-10-05
C++ 学习 ---cstdio 的源码学习分析 06- 关闭文件函数 fclose
stdio.h中定义了一系列文件访问函数(fopen,fclose,fflush,freopen,setbuf,setvbuf),接下来我们一起来分析一下fclose对应的源码实现。
2022-10-06
国庆策划 03|揭秘代码优化操作和栈保护机制
今天是国庆假期策划的第三期。我们来公布第一期主观题的答案,揭秘代码优化操作和栈保护机制。
2022-10-07
推荐阅读
低代码起势,程序员闷头开发的日子结束了
2023-04-14
3、yml 配置文件加载流程源码解析
2023-09-28
数字化驱动,低代码引领:探索数智时代的开发新纪元!
2023-06-28
音视频开发 _ 获取媒体文件的详细信息
2023-04-21
37. 点对点调用案例搭建
2023-09-29
长安链源码分析之交易过程分析(8)
2022-10-25
9、源码阅读之 mapper 代理底层源码
2023-09-28
电子书
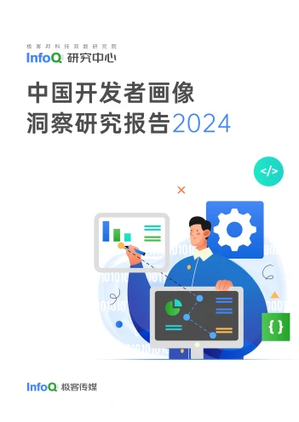
大厂实战PPT下载
换一换 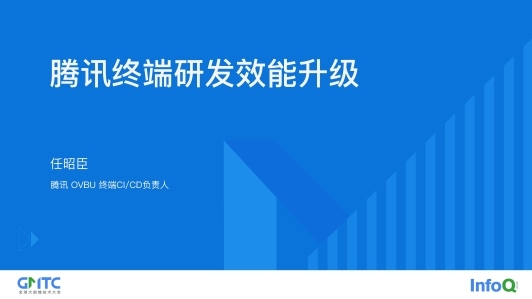
任昭臣 | 腾讯 OVBU 终端 CI/CD 负责人
刘育良 | 快手 AI 平台大模型训练负责人
汪成坤 | 众安科技 研发质量架构师
评论